Your first Python program: File I/O
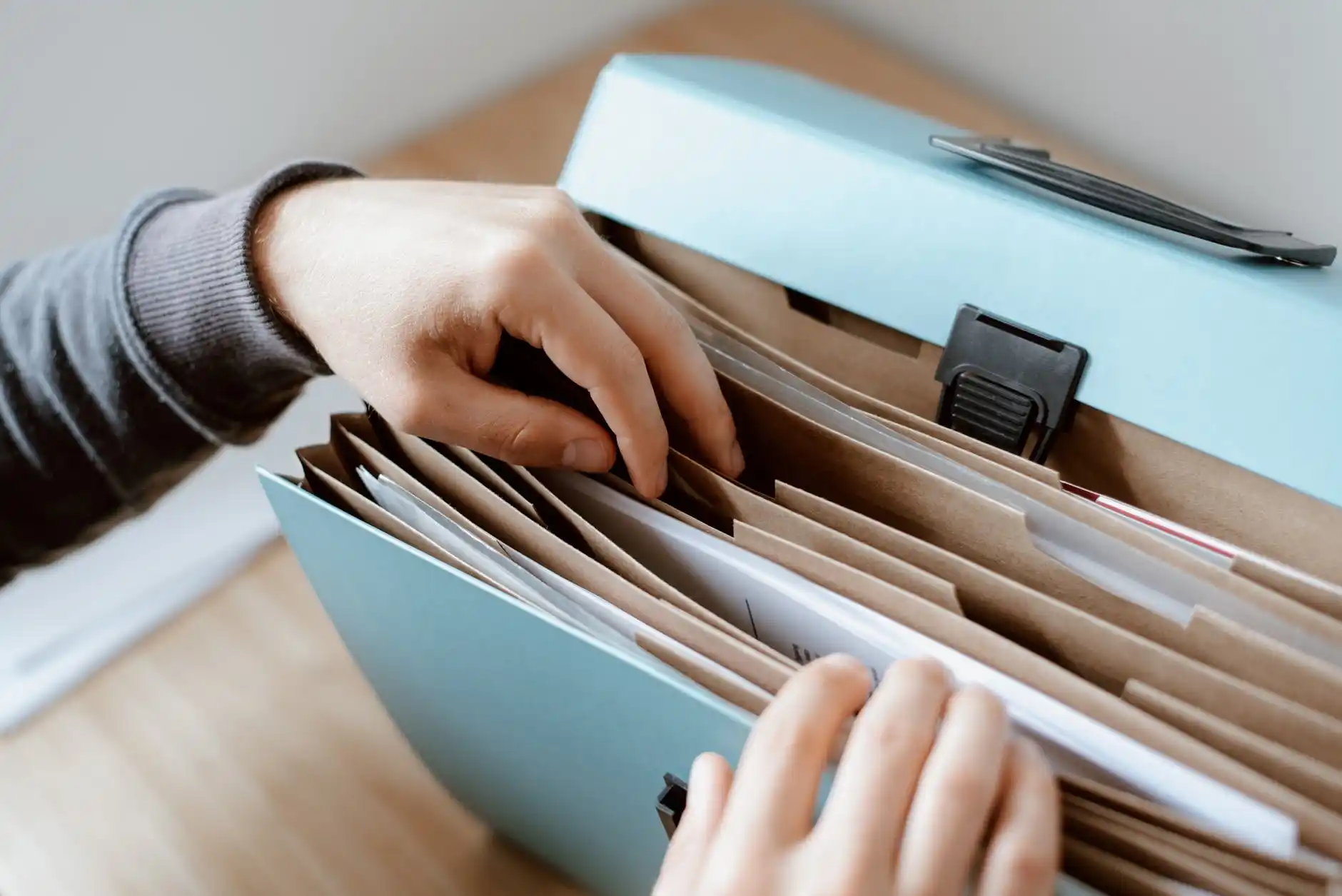
In the last post, we looked at setting up Python and interacting with it through a terminal. While running Python interactively is great to try a few commands, most of the time you will want a script that you can execute many time without rewriting the procedure.
For our first script, let’s take a problem that you will face a lot:
reading from and writing to files (developers call this “file I/O”).
Our toy problem will be to create a file and script that count how often the file has been read by the script.
For this, first create a file counter.txt
in a directory of your choice.
Write a single zero in it and save.
Now let’s look at the Python part.
To write a script, you will typically want an IDE (integrated development environment). We will look at different IDEs in another post. For now, just open the IDE shipped with your Python installation which is called “IDLE”. You will see an interactive terminal open up:
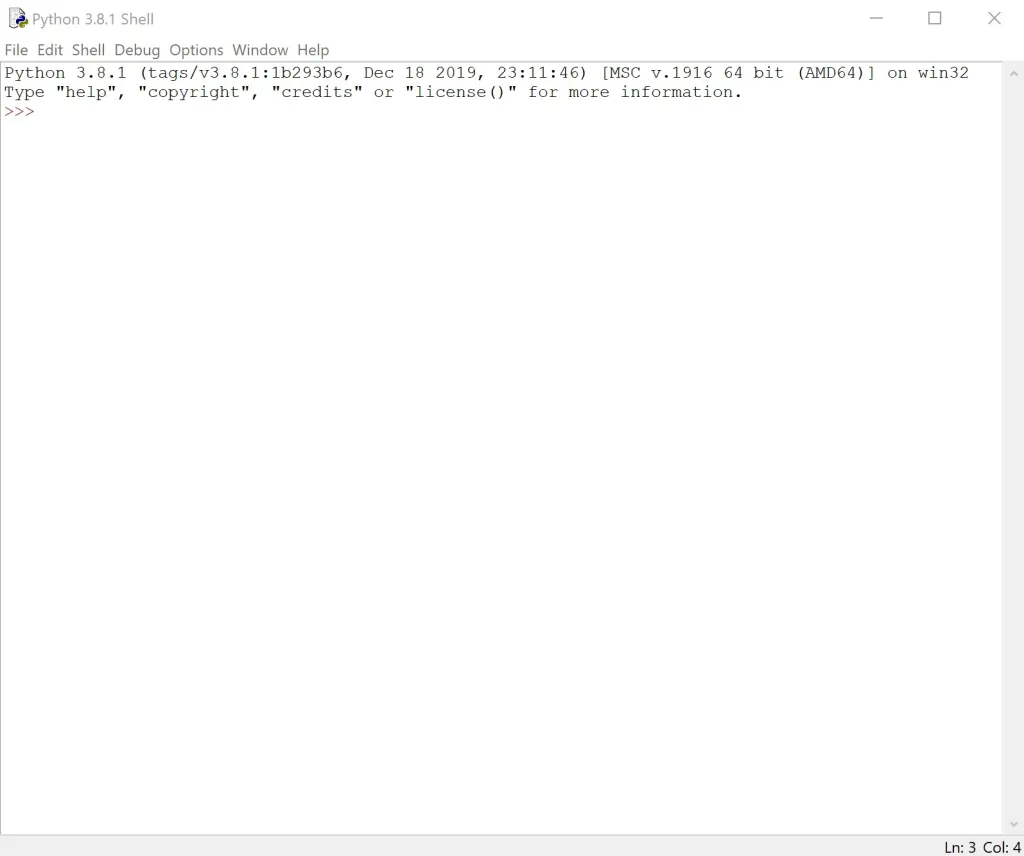
With File > New File...
, open a second window.
This is just a plain file and if you type there, you will not have any interactive behavior.
This is where we write the actual program.
Copy this code to your file (explanation below):
with open('counter.txt', 'r') as file: # open file for reading
n = int(file.read()) # read the content of the file into variable "n". Ensure type is int.
print(n) # print the content of "n"
n += 1 # add 1 to "n"
with open('counter.txt', 'w') as file: # open file for writing (this deletes previous content)
file.write(str(n)) # write the content of "n" (which was increased by 1) to the file
Before we inspect the code, let’s see it in action!
Save the code to the same directory as the file counter.txt
.
(On Windows, make sure the file ends in .py
, e.g. counter.py
).
Now click Run > Run Module.
You will be thrown back into the interactive window where a zero pops up.
Run the script a few more times and you will see what it does.
For the explanation:
- The first line of our code opens the file
counter.txt
for reading (hence the'r'
). The with statement conveniently takes care of closing the file for us after execution of the indented block as well. - In the indented line,
file.read()
is a method that actually fetches the content of the file and saves it in a variablen
. We enclosefile.read()
inint()
to enforce this to be an integer (as opposed to a string that we will typically receive when reading a text file) - With
print(n)
, we display the content ofn
in the interactive output - The next line is shorthand for
n = n + 1
- To edit
counter.txt
, we again use thewith open(...) as file
syntax. Note how we use'w'
for writing this time. Further note that when opening a file with'w'
, it deletes any previous content. - Similar to the
read()
part, we must explicitly state the content to be written to the open file withfile.write()
. We usestr(n)
, thus transforming the content ofn
(an integer as you might recall) into a string
And that’s it! If you are absolutely new to Python, I invite you to note a few more little things regarding the syntax:
- Note how strings are enclosed in quotes
''
. I use single quotes here, but double quotes""
work as well. - Note how I wrote comments into the script, all starting with
#
. Adding comments to your work is an important habit, not only so that other people understand it, but often even more so that you can understand what you coded three months ago
Congrats! You wrote (and hopefully understood) your first Python program!